[Dibi](https://dibiphp.com) - smart database layer for PHP [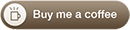](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=9XXL5ZJHAYQUN)
=========================================================
[](https://packagist.org/packages/dibi/dibi)
[](https://travis-ci.org/dg/dibi)
[](https://ci.appveyor.com/project/dg/dibi/branch/master)
[](https://github.com/dg/dibi/releases)
[](https://github.com/dg/dibi/blob/master/license.md)
Database access functions in PHP are not standardised. This library
hides the differences between them, and above all, it gives you a very handy interface.
Installation
============
The best way how to install Dibi is to [download a latest package](https://github.com/dg/dibi/releases) or use a Composer:
```bash
composer require dibi/dibi
```
The Dibi 3 requires PHP version 5.4.4 or newer (is compatible with PHP 7.0 and 7.1), version 4 requires PHP 7.1.
Examples
--------
Refer to the `examples` directory for examples. Dibi documentation is
available on the [homepage](https://dibiphp.com).
Connect to database:
```php
// connect to database (static way)
dibi::connect([
'driver' => 'mysql',
'host' => 'localhost',
'username' => 'root',
'password' => '***',
]);
// or object way; in all other examples use $connection-> instead of dibi::
$connection = new DibiConnection($options);
```
SELECT, INSERT, UPDATE
```php
dibi::query('SELECT * FROM users WHERE id = ?', $id);
$arr = [
'name' => 'John',
'is_admin' => true,
];
dibi::query('INSERT INTO users', $arr);
// INSERT INTO users (`name`, `is_admin`) VALUES ('John', 1)
dibi::query('UPDATE users SET', $arr, 'WHERE `id`=?', $x);
// UPDATE users SET `name`='John', `is_admin`=1 WHERE `id` = 123
dibi::query('UPDATE users SET', [
'title' => array('SHA1(?)', 'tajneheslo'),
]);
// UPDATE users SET 'title' = SHA1('tajneheslo')
```
Getting results
```php
$result = dibi::query('SELECT * FROM users');
$value = $result->fetchSingle(); // single value
$all = $result->fetchAll(); // all rows
$assoc = $result->fetchAssoc('id'); // all rows as associative array
$pairs = $result->fetchPairs('customerID', 'name'); // all rows as key => value pairs
// iterating
foreach ($result as $n => $row) {
print_r($row);
}
```
Modifiers for arrays:
```php
dibi::query('SELECT * FROM users WHERE %and', [
array('number > ?', 10),
array('number < ?', 100),
]);
// SELECT * FROM users WHERE (number > 10) AND (number < 100)
```
%and | | `[key]=val AND [key2]="val2" AND ...` |
%or | | `[key]=val OR [key2]="val2" OR ...` |
%a | assoc | `[key]=val, [key2]="val2", ...` |
%l %in | list | `(val, "val2", ...)` |
%v | values | `([key], [key2], ...) VALUES (val, "val2", ...)` |
%m | multivalues | `([key], [key2], ...) VALUES (val, "val2", ...), (val, "val2", ...), ...` |
%by | ordering | `[key] ASC, [key2] DESC ...` |
%n | identifiers | `[key], [key2] AS alias, ...` |
other | - | `val, val2, ...` |
Modifiers for LIKE
```php
dibi::query("SELECT * FROM table WHERE name LIKE %like~", $query);
```
%like~ | begins with |
%~like | ends with |
%~like~ | contains |
DateTime:
```php
dibi::query('UPDATE users SET', [
'time' => new DateTime,
]);
// UPDATE users SET ('2008-01-01 01:08:10')
```
Testing:
```php
echo dibi::$sql; // last SQL query
echo dibi::$elapsedTime;
echo dibi::$numOfQueries;
echo dibi::$totalTime;
```